AuboCaps
Teaching Pendant Plugin Development Guide
AuboCaps provides all necessary interfaces and configurations for plugin development in AUBO robot's AUBO Scope software. Users can develop plugins based on the information provided in this manual, integrate them into AUBO Scope software, and enhance the functionality of AUBO robots.
AUBO Scope Workflow
Plugins ultimately run on the teach pendant, so certain functionalities of plugins (primarily in program nodes) need to coordinate with the teach pendant software.
Within the AUBO Scope software architecture, there are mainly two components: the teach pendant and the controller. AUBO Scope software communicates with the robotic arm through scripts, following these general steps:
- Users perform online programming or configure relevant parameters;
- Programs or configured parameters are recorded in the teach pendant;
- The teach pendant generates script files;
- The teach pendant sends all script files to the controller;
- The controller generates robot control commands, sends them to the robotic arm, and executes the corresponding operations.
Core Tasks of Plugin Development
As inferred from the AUBO Scope workflow, the essence of plugin development is to provide scripts to the robotic arm controller through plugins. Within the teach pendant, scripts originate from installation nodes and program nodes. Therefore, the primary tasks of plugin development are to complete the installation node and program node modules of the plugin.
Installation Node
Installation nodes are mainly responsible for configuring various parameters of the plugin and saving these configurations. Similar to configuring basic parameters of a camera, such as exposure time and camera intrinsics, installation nodes handle setting these parameters.
It's important to note that installation nodes are instantiated only once in the program. This is akin to installing other software applications only once. Since parameters are a global concept, all subsequent physical operations are based on these parameters, necessitating instantiation only once. Additionally, this feature can significantly enhance operational efficiency.
Program Node
Program nodes primarily generate scripts based on parameters to execute specific functions. For instance, when the robotic arm moves to a certain point, a program node at that point might be responsible for opening the gripper. Similarly, when the robotic arm moves to another point, another program node might be responsible for closing the gripper.
Plugin Template Structure
API
AUBO Scope provides API interfaces that users can directly call during plugin development, reducing development time. Based on the usage restrictions of different APIs in installation nodes and program nodes, APIs can be divided into two main categories: domain_api and aubo_cpa_api. Within domain_api, system_api, user_interface_api, and application_api can be used in both installation nodes and program nodes. The program_api in aubo_cpa_api is only usable in program nodes, while installation_api is exclusive to installation nodes. For detailed descriptions of domain_api and aubo_cpa_api, refer to the diagram below.
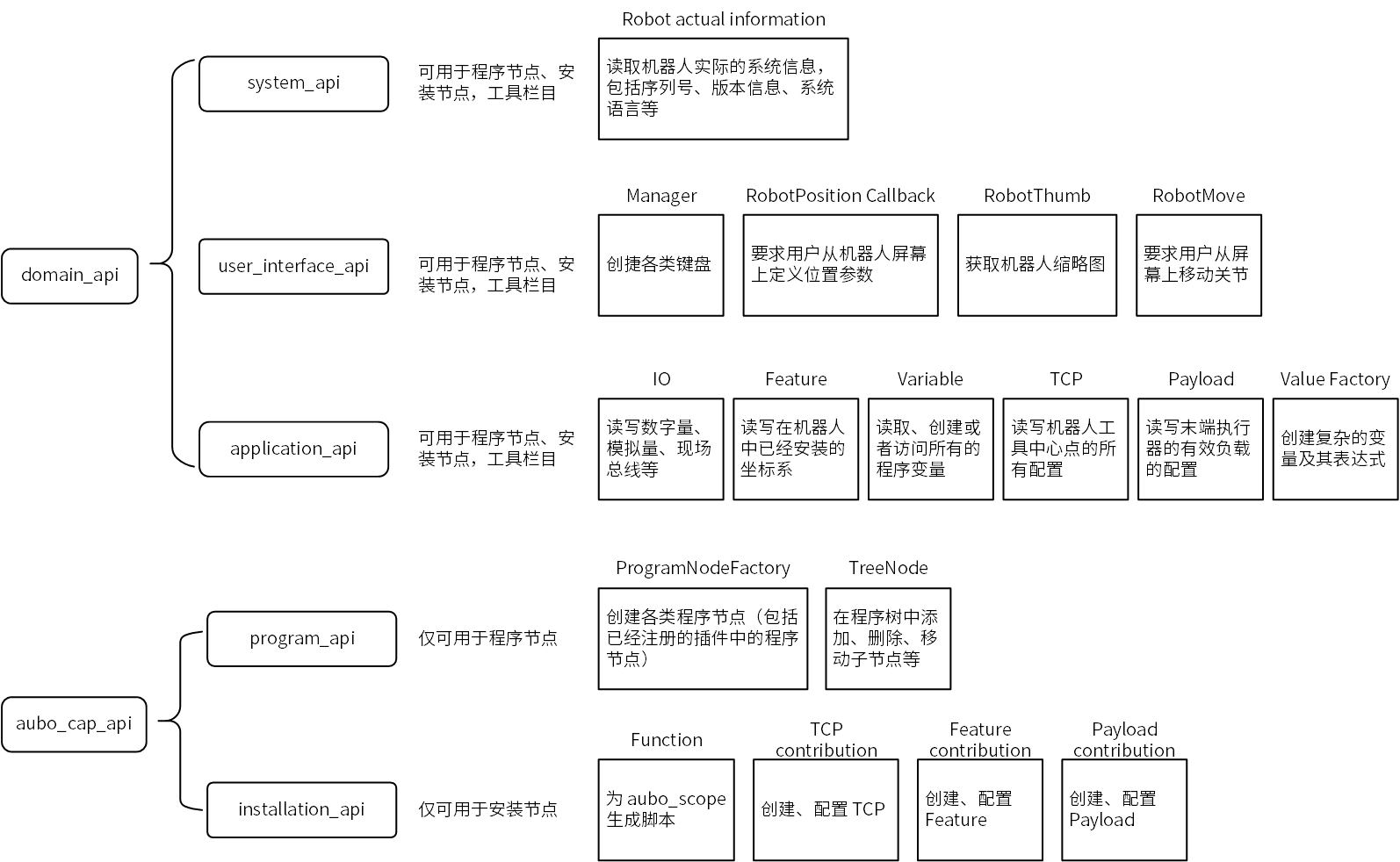
注意
For detailed information on each interface, please refer to the Appendix: aubo_caps API
API Usage Overview
In the template, both installation nodes and program nodes include three classes: service, contribution, and view. The service class in installation nodes serves to create contribution and view classes. The contribution class contains data-related content, while the view class contains interface-related content. Details are as follows:
Interfaces in the service class of installation nodes:
getTitle
、getIcon
、createView
、configureContribution
、createInstallationNode
Interfaces in the service class of program nodes:
getTitle
、getIcon
、getId
、createView
、configureContribution
、createNode
api_provider_ interface in the contribution class of installation nodes:
systemApi
、userInterfaceApi
、installationApi
api_provider_ interface in the contribution class of program nodes:
systemApi
、userInterfaceApi
、programApi
view_api_ interface in both installation nodes and program nodes' view classes:
systemApi
、userInterfaceApi
Accessing data using DataModel:
- Configuration Data is generally stored in the [/root/arcs_ws/program] folder. When aubo_scope starts, the saved configuration data is loaded into the DataModel.
- The interface DataModelPtr model_{ nullptr } provides a mechanism in both installation and program environments. This interface allows storing data items into the DataModel (currently supporting only specific data types, details in Appendix: aubo_caps API).
- Usage of the interface DataModelPtr model_{ nullptr }:
- Add the header file
#include "aubo_caps++/meta_type.h"
. - Add
DECLARE_ATTR
in the private section of the contribution class.
- Add the header file